Warm Ups:
import { Component } from '@angular/core';
export class Technology {
constructor(public id: number, public name: string,
public power: string, public description: string) { }
}
@Component({
selector: 'app-technology-form',
template: `
{{ result }}
`
})
export class TechnologyFormComponent {
result = '';
private myTechnology = new Technology( 42, 'Angular 5',
'Single Page Applications',
'One Framework. Mobile & desktop.');
constructor() {
this.result = 'My technology is called ' + this.myTechnology.name;
}
}
import { Component } from '@angular/core';
export class Technology {
constructor(public id: number, public name: string,
public power: string, public description?: string) { }
}
@Component({
selector: 'app-technology-form',
template: `
<div class="container">
<div [hidden]="submitted">
<h1>Technology Form</h1>
<form (ngSubmit)="onSubmit()" #technologyForm="ngForm">
<div class="form-group">
<label for="name">Name</label>
<input type="text" class="form-control" id="name"
[(ngModel)]="model.name" name="name" required
#name="ngModel">
<div [hidden]="name.valid || name.pristine" class="alert alert-danger">
Name is required
</div>
</div>
<div class="form-group">
<label for="description">Description</label>
<input type="text" class="form-control" id="description"
[(ngModel)]="model.description" name="description">
</div>
<div class="form-group">
<label for="power">Technology Power</label>
<select class="form-control" id="power" required
[(ngModel)]="model.power" name="power">
<option *ngFor="let pow of powers" [value]="pow">{{ pow }}</option>
</select>
</div>
<button type="submit" class="btn btn-success"
[disabled]="!technologyForm.form.valid"
>Submit</button>
<button type="button" class="btn btn-default"
(click)="newTechnology(); technologyForm.reset();">
New Technology</button>
</form>
</div>
<div [hidden]="!submitted">
<h2>You submitted the following</h2>
<div class="row">
<div class="col-xs-3">Name:</div>
<div class="col-xs-9 pull-left">{{ model.name }}</div>
</div>
<div class="row">
<div class="col-xs-3">Description:</div>
<div class="col-xs-9 pull-left">{{ model.description }}</div>
</div>
<div class="row">
<div class="col-xs-3">Power:</div>
<div class="col-xs-9 pull-left">{{ model.power }}</div>
</div>
<br>
<button class="btn btn-primary" (click)="submitted=false">Edit</button>
</div>
</div>
`,
styles: [`
.ng-valid[required], .ng-valid.required {
border-left: 5px solid #42A948;
}
.ng-invalid:not(form) {
border-left: 5px solid #a94442;
}
`]
})
export class TechnologyFormComponent {
powers = ['Data Binding', 'Routing', 'Animations', 'Dependency Injection'];
model = new Technology( 42, 'Angular 5', this.powers[0],
'One Framework. Mobile & desktop.');
submitted = false;
newTechnology() { this.model = new Technology(100, '', ''); }
onSubmit() { this.submitted = true; }
get diagnostic() { return JSON.stringify(this.model); }
}
AppComponent:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<h1>{{ title }}</h1>
<div class="left">
<app-technology-form></app-technology-form>
</div>
<div class="right">
<img [src]="imageUrl" class="img-round" alt="princess image">
</div>
`,
styles: [`h1 { font-weight: bold; }`]
})
export class AppComponent {
title = 'Tour of Technologies';
imageUrl = '../assets/polymer3.jpg';
}
Let's have a look at this beauty in our Chromium Browser:
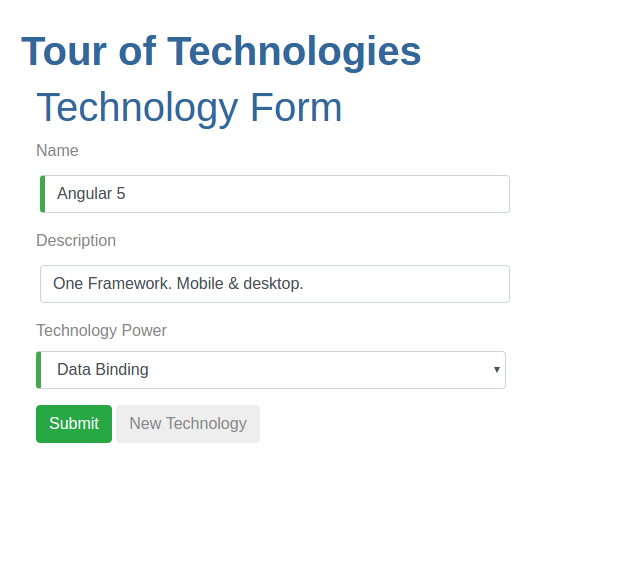
import { Component } from '@angular/core';
@Component({
selector: 'app-technology-form',
template: `
<form #technologyForm="ngForm" (ngSubmit)="submit(technologyForm)">
<div class="form-group">
<label for="name">Name:</label>
<input
required
minlength="5"
maxlength="20"
pattern="Angular 5"
ngModel
name="name"
#name="ngModel"
id="name"
type="text"
class="form-control">
<div class="alert alert-danger"
*ngIf="name.touched && !name.valid">
<div *ngIf="name.errors.required">
Name is required.
</div>
<div *ngIf="name.errors.minlength">
Name should be a minimum of {{ name.errors.minlength.requiredLength }} characters.
</div>
<div *ngIf="name.errors.pattern">
Name doesn't match pattern.
</div>
</div>
</div>
<div class="form-group">
<label for="description">Description:</label>
<textarea ngModel
name="description"
id="description"
cols="30"
rows="5"
type="text"
class="form-control">
</textarea>
</div>
<div class="checkbox">
<label>Subscribe to mailing list
<input type="checkbox" ngModel name="isSubscribed">
</label>
</div>
<div class="form-group">
<label for="contactMethod">Contact Method</label>
<select ngModel name="contactMethod" id="contactMethod" class="form-control">
<option value="">Select</option>
<option *ngFor="let method of contactMethods" [ngValue]="method">{{ method.name }}</option>
</select>
</div>
<p>
{{ technologyForm.value | json }}
</p>
<button class="btn btn-danger" [disabled]="!technologyForm.valid">Submit</button>
</form>
`,
styles: [`
.form-control.ng-touched.ng-invalid {
border-left: 5px solid red;
}
.form-control.ng-pristine,ng-untouched.ng-invalid {
border-left: 5px solid green;
}
`]
})
export class TechnologyFormComponent {
contactMethods = [
{ id: 1, name: 'Email' },
{ id: 2, name: 'Google Hangouts' },
{ id: 3, name: 'Android Mobile' },
{ id: 4, name: 'WhatsApp' }
];
submit(technologyForm) {
console.log(technologyForm.value);
}
}
Let's have a look at this beauty in our Chromium Web Browser:
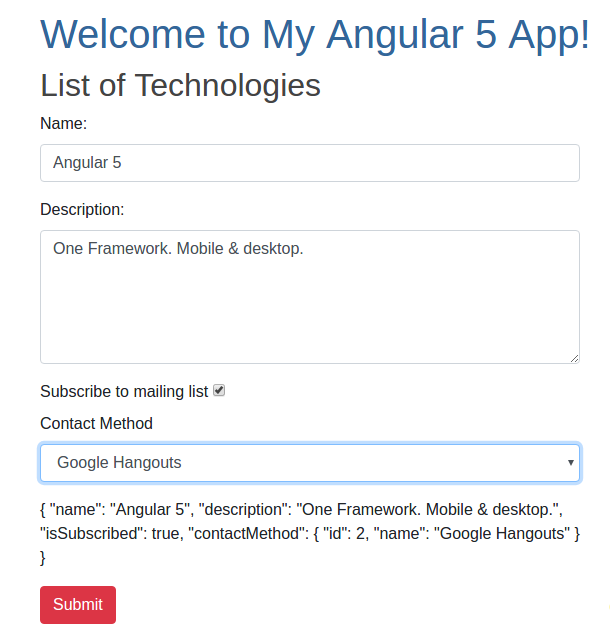